Using PowerShell to Automate Operational Tasks⚙️
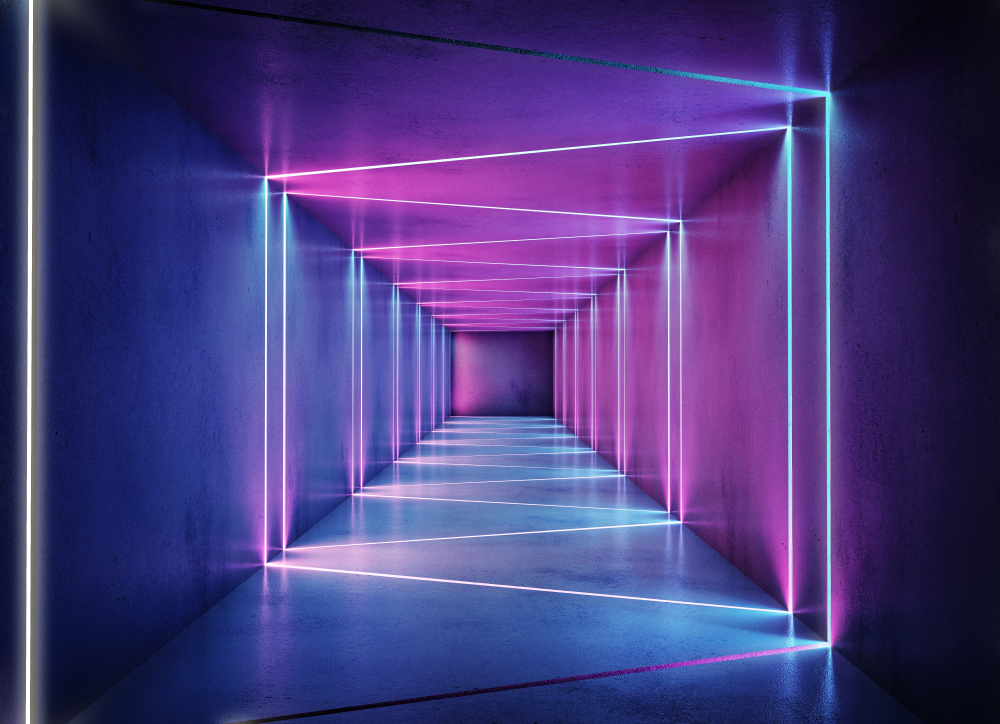
Some frequently used, time-saving commands/tips on Linux, PowerShell, Excel, Windows, etc.
SHELLing out tips which have saved me time
- Add Print Server
- Kaspersky Virus Scanning
- Verify Active Users from CSV File
- Check if Distribution Group is Nested
- Get AD User Attributes for a Set of Users and return a Single Table
Add Print Server
# Add Print Server based on the Geographic Location
$NYPrinter = "\\NYPRINT\SmartPrint"
$AustinPrinter = "\\AUSPRINT\SmartPrint"
$publicIP = cmd /c "curl ifconfig.me"
Write-Host $nyIP
If ($publicIP -eq "X.X.X.X") {
Write-Host "NYC connection!"
Add-Printer -ConnectionName $NYPrinter -ErrorAction SilentlyContinue
}
ElseIf ($publicIP -eq "Y.Y.Y.Y"){
Write-Host "Austin connection!"
Add-Printer -ConnectionName $AustinPrinter -ErrorAction SilentlyContinue
}
Kaspersky Virus Scanning
# Download Kaspersky's Virus Scanner, Initiate a Background Scan against All Volumes, then delete the Virus Scanner EXE
curl https://devbuilds.s.kaspersky-labs.com/devbuilds/KVRT/latest/full/KVRT.exe --output %UserProfile%\Desktop\KVRT.exe
start %UserProfile%\Desktop\KVRT.exe -accepteula -d C:\temp -allvolumes -silent
DEL %UserProfile%\Desktop\KVRT.exe
Verify Active Users from CSV File
Import-Csv Names.csv -delimiter "," | ForEach-Object { $user = $_.usernames; Get-ADUser -Identity $user -Properties * | Select-Object Name, Enabled}
Check if Distribution Group is Nested
Install-Module -Name ExchangeOnlineManagement
Update-Module -Name ExchangeOnlineManagement
Connect-ExchangeOnline -UserPrincipalName username@domain.com
$allDistros = Get-DistributionGroup -ResultSize Unlimited
ForEach ($Distro in $allDistros) {
#Write-Host $Distro.GetType()
#Write-Host $Distro.ToString()
$DistributionGroupMembers = Get-DistributionGroupMember -identity $Distro.DistinguishedName | Select-Object -ExpandProperty Name | Out-String #$Distro.ToString()
#Write-Host $DistributionGroupMembers
If ($DistributionGroupMembers.Contains('NAMEOFDISTRO')){
Write-Host "GROUPA@domain.com is a member of $Distro"
}
}
Disconnect-ExchangeOnline -Confirm:$false
Get AD User Attributes for a Set of Users and return a Single Table
$array = @(
'username'
)
$Fullresult=@()
foreach ($upn in $array) {
Try{
$user = Get-ADUser -identity $upn -properties PwdLastSet, Enabled, DisplayName, Title
#Get-ADUser -Filter {samAccountName -eq $upn} -Property DisplayName | Select-Object DisplayName #filter by custom attribute
$userPropertiesArray = [PSCustomObject]@{
Name = $user.Name
DisplayName = $user.DisplayName
EmailAddress = $user.EmailAddress
Title = $user.Title
PwdLastSet = [DateTime]::FromFileTime($user.PwdLastSet)
Enabled = $user.Enabled
}
}Catch{
$userPropertiesArray = [PSCustomObject]@{
Name = $upn
DisplayName = ''
EmailAddress = ''
Title = ''
PwdLastSet = ''
Enabled = ''
}
}
$Fullresult+=$userPropertiesArray
}
return $Fullresult | FT